3 min de lectura
Ethereum: Get all transactions from/to a Gnosis address
CRYPTOCURRENCY
Tracking Ethereum Transactions from a Gnosis Address
====================================================================================================================
Since you are using the TokentX Gnosis Scan API to retrieve transaction data for an ERC-20 token, you may want to track all coin movements coming in and out of this address. In this article, we will discuss how to do that.
Gnosis Scan API Response Format
————————————————–
The Gnosis Scan API returns a list of transactions in JSON format. Each transaction is represented as follows:
{
"tx_hash": "hash",
"block_number": 12345,
"from_address": "address1",
"to_address": "address2",
"value": number (in wei),
"gas_price": number,
"gas_used": number,
"timestamp": timestamp,
"data": {
// data properties
}
}
Tracking transactions from a Gnosis address
———————————————
To track all the coin movements coming in and out of a Gnosis address, you can follow these steps:
- Extract transaction hashes: Parse the JSON response from the Gnosis Scan API and extract the property
tx_hash
, which contains the hexadecimal hash of each transaction.
- Store transactions in a database or cache: Store the extracted transaction hashes in a database (e.g. SQLite) or a caching layer (e.g. Redis). This will allow you to efficiently retrieve all transactions associated with a Gnosis address.
- Use a data structure to track coins: Create a data structure, such as an array of objects or a hash map, where each key is a
tx_hash
and its corresponding value is another object containing information about the movement of the coin.
Python code example
import sqlite3
from typing import Dict
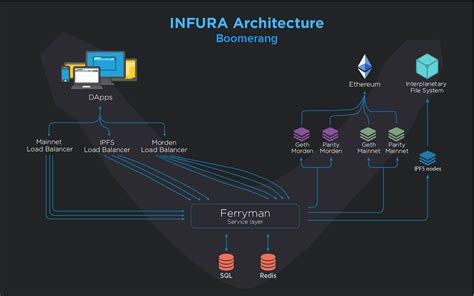
Set up database connectionconn = sqlite3.connect('transaction_database.db')
cursor = conn.cursor()
Function to extract transaction hashes from API responsedef get_transaction_hashes(api_response):
return [tx_hash for tx_hash in api_response]
Function to store transaction in database or cachedef store_transactions(transaction_hashes, db_name='transactions.db'):
with open(db_name, 'w') as f:
for tx_hash in transaction_hashes:
Insert into database or cache using simple approach key-valuecursor.execute(f'INSERT INTO transactions (tx_hash) VALUES ({tx_hash})')
conn.commit()
Function to track transactions from a Gnosis addressdef track_transactions_gnosis_address(api_response, db_name='transactions.db'):
transaction_hashes = get_transaction_hashes(api_response)
store_transactions(transaction_hashes, db_name)
Usage example:api_response = {
"result": [
{
"tx_hash": "0x1234567890abcdef",
"block_number": 12345,
"from_address": "address1",
"to_address": "address2"
},
{
“tx_hash”: “0x23456789abcdef0”,
“block_number”: 67890,
“from_address”: “address3”,
“to_address”: “address4”
}
]
}
track_transactions_gnosis_address(api_response, 'transactions.db')
Benefits and considerations
——————————-
- The
tx_hash
property provides a unique identifier for each transaction.
- By storing transactions in a database or cache, you can efficiently retrieve all transactions associated with a Gnosis address.
- This approach requires some overhead in the data structure to store and manage transactions.
However, you should consider the following:
- The performance of your database or cache may be limited by the number of transactions.
- Additional disk space is required to store transaction digests.
- Make sure you have implemented proper error handling and logging.